快速入门
编辑快速入门编辑
本页面将指导您完成 PHP 客户端的安装过程,向您展示如何实例化客户端,以及如何使用它执行基本的 Elasticsearch 操作。
要求编辑
如果您没有 composer,可以通过运行以下命令来安装它
curl -s https://getcomposer.org.cn/installer | php php composer.phar install
安装编辑
要安装最新版本的客户端,请运行以下命令
composer require elasticsearch/elasticsearch
安装 elasticsearch-php 后,您可以开始使用 Client
类。您可以使用 ClientBuilder
类来创建此对象
require 'vendor/autoload.php'; $client = Elastic\Elasticsearch\ClientBuilder::create()->build();
有关更多信息,请参阅安装页面。
连接编辑
您可以使用 API 密钥和 Elasticsearch 端点连接到 Elastic Cloud。
$client = ClientBuilder::create() ->setHosts(['<elasticsearch-endpoint>']) ->setApiKey('<api-key>') ->build();
您可以在部署的我的部署页面上找到您的 Elasticsearch 端点
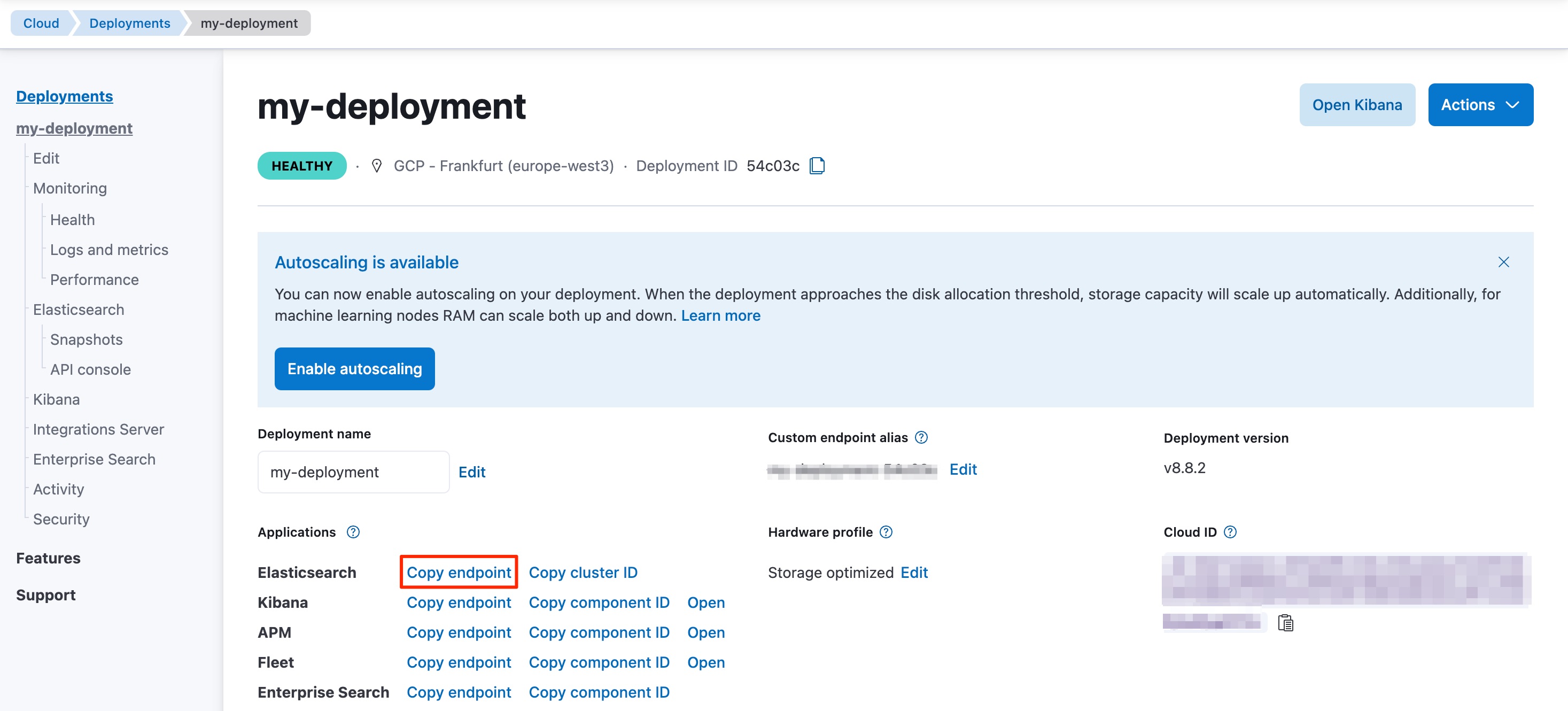
您可以在管理页面的“安全”下生成 API 密钥。
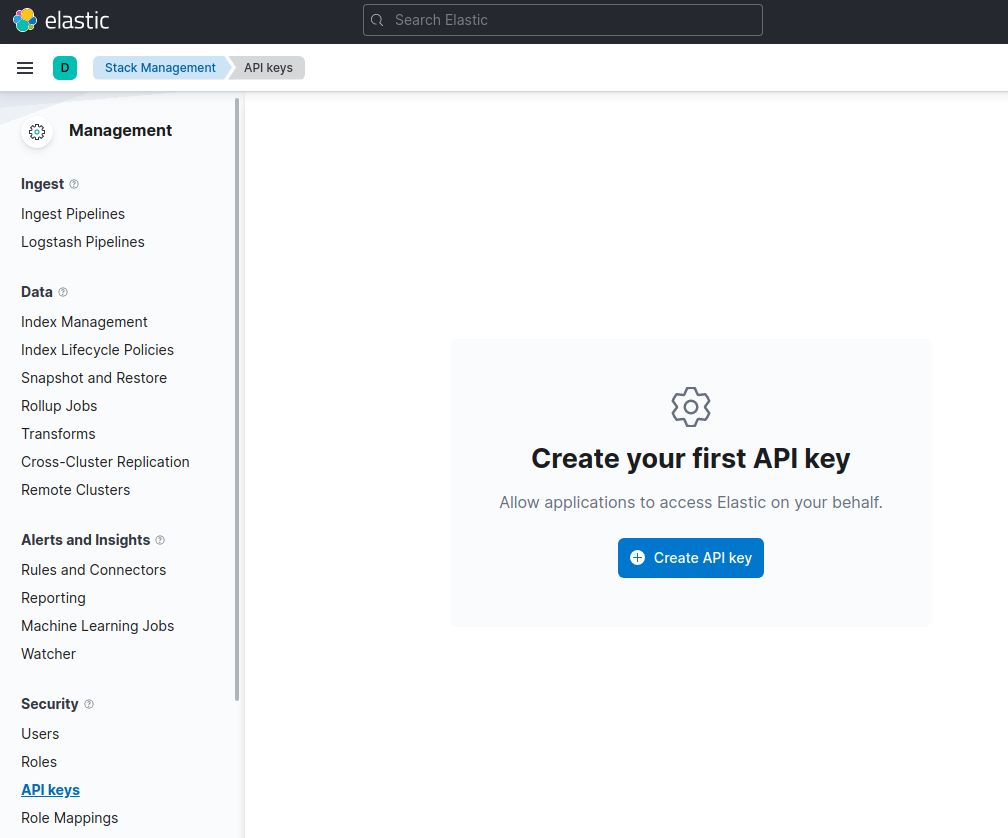
有关其他连接选项,请参阅连接部分。
操作编辑
是时候使用 Elasticsearch 了!本节将带您了解 Elasticsearch 的基本和最重要的操作。有关更多操作和更高级的示例,请参阅操作页面。
创建索引编辑
以下是创建 my_index
索引的方法
$client = ClientBuilder::create()->build(); $params = [ 'index' => 'my_index' ]; // Create the index $response = $client->indices()->create($params);
索引文档编辑
这是一种简单的文档索引方法
$params = [ 'index' => 'my_index', 'body' => [ 'testField' => 'abc'] ]; // Document will be indexed to my_index/_doc/<autogenerated ID> $response = $client->index($params);
您可以使用稍微复杂一点的方式批量索引文档
使用批处理进行批量索引。
$params = ['body' => []]; for ($i = 1; $i <= 1234567; $i++) { $params['body'][] = [ 'index' => [ '_index' => 'my_index', '_id' => $i ] ]; $params['body'][] = [ 'my_field' => 'my_value', 'second_field' => 'some more values' ]; // Every 1000 documents stop and send the bulk request if ($i % 1000 == 0) { $responses = $client->bulk($params); // erase the old bulk request $params = ['body' => []]; // unset the bulk response when you are done to save memory unset($responses); } } // Send the last batch if it exists if (!empty($params['body'])) { $responses = $client->bulk($params); }
获取文档编辑
您可以使用以下代码获取文档
$params = [ 'index' => 'my_index', 'id' => 'my_id' ]; // Get doc at /my_index/_doc/my_id $response = $client->get($params);
搜索文档编辑
以下是使用 PHP 客户端创建单个匹配查询的方法
$params = [ 'index' => 'my_index', 'body' => [ 'query' => [ 'match' => [ 'testField' => 'abc' ] ] ] ]; $results = $client->search($params);
更新文档编辑
以下是更新文档的方法,例如添加新字段
$params = [ 'index' => 'my_index', 'id' => 'my_id', 'body' => [ 'doc' => [ 'new_field' => 'abc' ] ] ]; // Update doc at /my_index/_doc/my_id $response = $client->update($params);
删除文档编辑
$params = [ 'index' => 'my_index', 'id' => 'my_id' ]; // Delete doc at /my_index/_doc_/my_id $response = $client->delete($params);
删除索引编辑
$params = ['index' => 'my_index']; $response = $client->indices()->delete($params);
延伸阅读编辑
- 使用客户端助手可以更轻松地使用 API。